Update Packages in Python Poetry
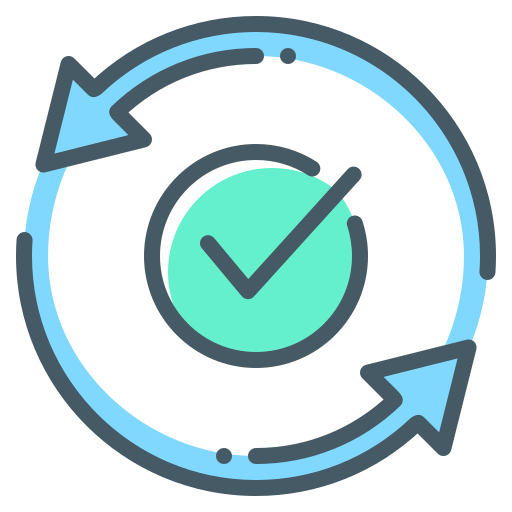
This tutorial will discuss the steps on how to safely update packages in Python Poetry.
If you have not used Poetry before or you need to install this in your project, please read first my tutorial about Python Poetry Cheat Sheet.
Verify Outdated Packages in Poetry
First step is to find out what packages are currently installed in your system. Then, find the ones that are outdated. You can easily show this by running a command poetry show
.
poetry show -l
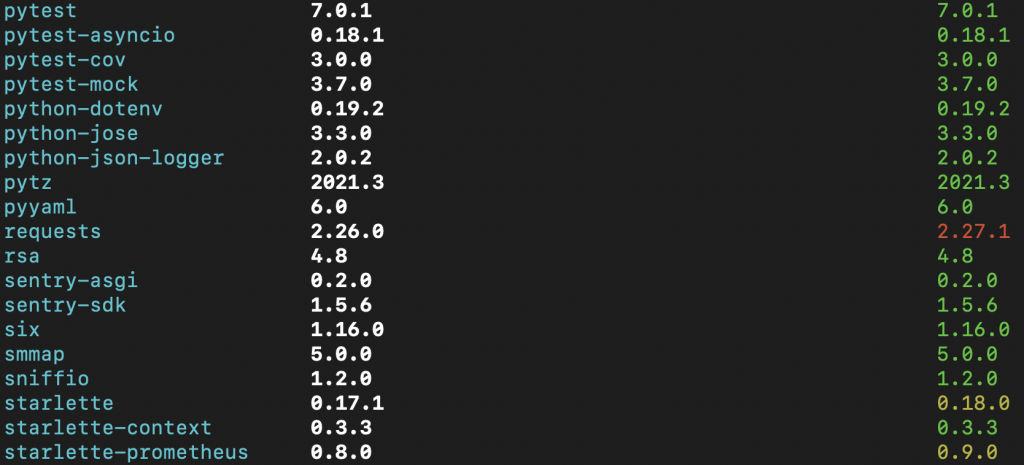
The -l
flag will include the latest version available for each packages installed in your system. You can see at the second column the current version of your package. And at the third column is the latest version. You will also notice that the color is green if your package is up to date, yellow and red if there is an available update.
It sure looks like a daunting task updating your system at a first glance. With all the shown packages, how do we know where to start? Really, most of the listed packages from the command above are just chain of dependencies. Now, remember that Poetry keeps a master list of all the packages that you have explicitly installed. That file is pyproject.toml
. My advise is to look at this file for starter.
Update Packages in Poetry
Poetry provides a command poetry update
for this task. Although, the version update varies on the semantic versioning of your packages. For example , a ^
caret would only update to the latest minor version.
If you need Poetry to update to a specific version, you can run a command poetry add
, passing the package name with the version as parameter.
poetry add "uvicorn==0.17.5"
(Or if your package accept extra parameters during installation)
poetry add "uvicorn[standard]==0.17.5"
You might be wondering why are we using poetry add
here? Well, Poetry is smart enough to detect if the package already exists in your system. It does not mean that it will keep two different versions of the package. But instead, it will replace the old version with the new one. At the same time, by running the said command, it will also update all its chain of dependencies. You will also notice that these changes are also reflected in the files pyproject.toml
and poetry.lock
. Now we’re rolling!
Tips: After you update individually the packages in pyproject.toml
, run again a mass update. This will allow poetry to check and update the remaining child dependencies.
poetry update
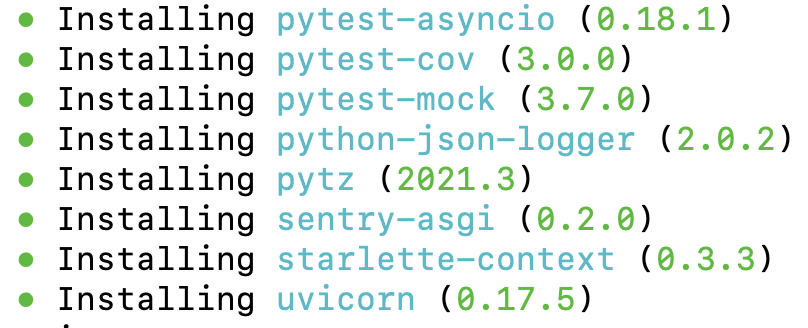
Which Package Depends to What?
When you install a package in your system, most of the time the package itself comes with its own dependencies. Poetry has a way to list the dependencies as a tree using again poetry show
but this time with a different flag.
poetry show -t
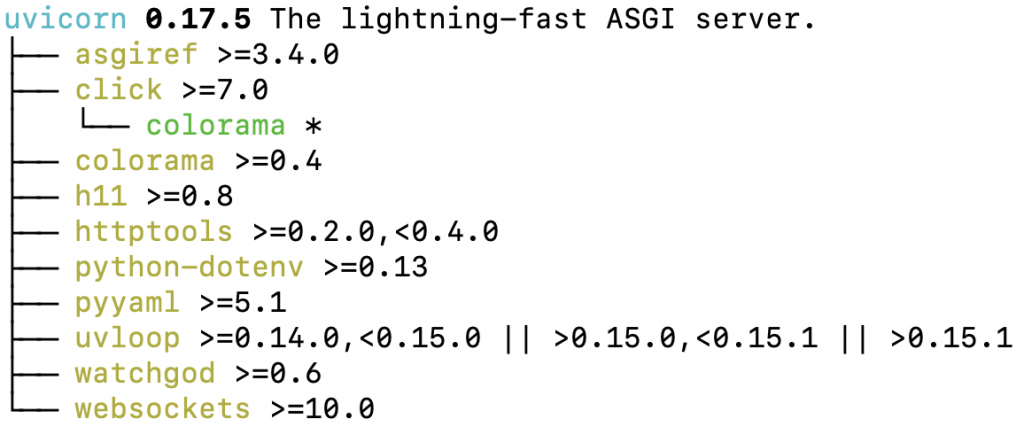
I advise that you look at the result of this command in your system. Sometimes you might be wondering why you are getting an error or it’s not installing any packages when updating to the latest version. The reason could be is that one or more of your packages depends to a specific version of another package. For example above, uvicorn
depends on httptools
version greater than or equals to 0.2.0
but less than 0.4.0
. Also, to avoid breaking changes in your system, you should know up to what version you are allowed to upgrade a package.
Poetry SolverProblemError during update
Because project-x depends on both mypy (0.931) and mypy (0.910), version solving failed.
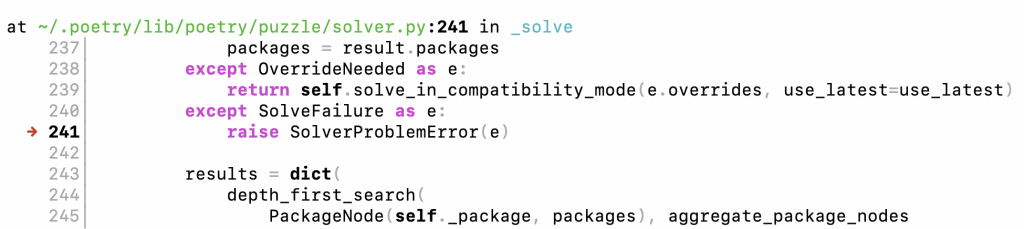
This error happens when you try to update a package that is a dependency in development without passing the --dev
flag. If you remember, without this flag, Poetry will add the package in tool.poetry.dependencies
section of pyproject.toml
which is the list of packages that gets deployed in production use. If the package that you are trying to update is in tool.poetry.dev-dependencies
section, make sure to add the flag.
poetry add "mypy==0.931" --dev
Poetry EnvCommand / FileNotFoundError Error during update
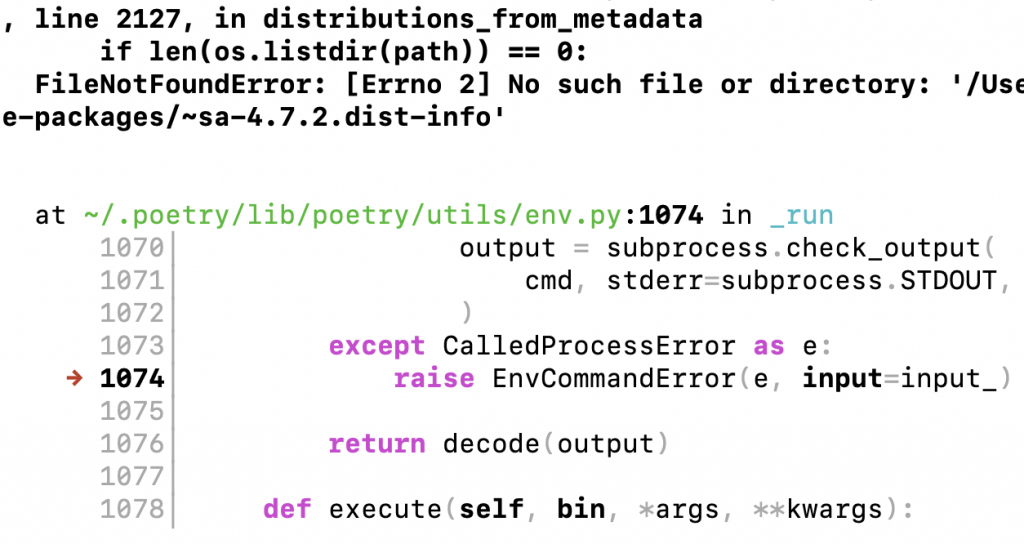
While/After updating packages in my system, I encountered some weird error saying “No such file or directory”. After some googling, I found a discussion about this error years ago. Some says it could be related to the virtual environment. But to be honest, these errors are still cryptic to me. Nevertheless, I wanted to share a possible fix that works for me (try at your own risk).
First, I make sure that my lock file poetry.lock is updated.
poetry lock
Second, I try to run poetry update
again. If you still get an error, it might be a good idea to rebuild your virtual environment. Make sure to update your lock file first.
poetry env list --full-path poetry env remove rex-XRBsn-py3.8 poetry env use $(which python3) source $(dirname $(poetry run which python3))/activate
- The first command is for listing your current virtual environment.
- Once you get the name of your environment, the second command is for removing this.
- The third command is for creating a new virtual environment.
- And finally, activating your new environment again.
Once you have a brand new environment, run an install.
poetry install
Recap of all the commands for updating packages in Python Poetry
For quick reference, here is a recap of all the commands I mentioned in this tutorial Update packages in Python Poetry.
poetry show -l poetry show -t poetry add "package==version" poetry add "package[extra]==version" poetry add "package==version" --dev poetry update poetry env list --full-path poetry env remove name-of-env poetry env use $(which python3) source $(dirname $(poetry run which python3))/activate