Getting started with Maven Cucumber JUnit and IntelliJ
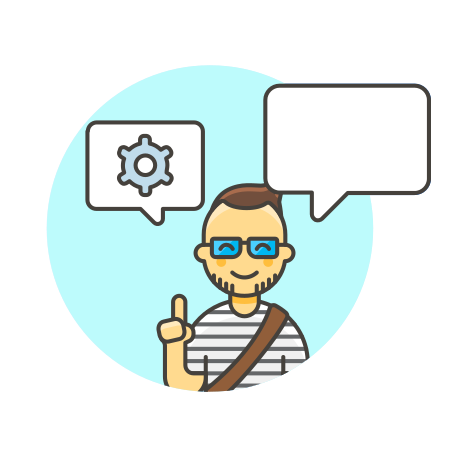
This tutorial will focus on the installation of Maven, Cucumber, JUnit and IntelliJ and other plugins to get you started writing your test suite in Java. This will not discuss theories and on how to write tests in Java.
How to install AdoptOpenJDK on MacOS
Add AdoptOpenJDK/openjdk in your Third-Party-Repositories (tap). Go to their GitHub for more versions of AdoptOpenJDK.
brew tap AdoptOpenJDK/openjdk
Install the specific version of OpenJDK
brew install --cask adoptopenjdk11
Check if you’ve successfully added OpenJDK in your Third-Party-Repositories
brew list --full-name
List all installed JDK.
/usr/libexec/java_home -V
Check the current version of your JDK
java -version
To support multiple version of JDK, copy the following line of commands in your .bash_profile
. The alias java11
is a quick way to reference an export
command to change the version assigned in JAVA_HOME
environment variable.
export JAVA_11_HOME=$(/usr/libexec/java_home -v 11.0.11) export JAVA_8_HOME=$(/usr/libexec/java_home -v 1.8.0_242) alias java11="export JAVA_HOME=$JAVA_11_HOME" alias java8="export JAVA_HOME=$JAVA_8_HOME"
How to install IntelliJ and plugins for Maven, Cucumber, Gherkin and JUnit
Download and install IntelliJ. For this example, I have installed the Community version of IntelliJ. For more IntelliJ configurations and on how to install this on Windows, please read my tutorial about “Install Amazon Corretto and IntelliJ IDEA in Windows“.
To add the plugins for Maven, Cucumber for Java and Gherkin go to IntelliJ IDEA > Preferences > Plugins. These plugins will allow you to generate your Maven project and start writing your Cucumber feature files.
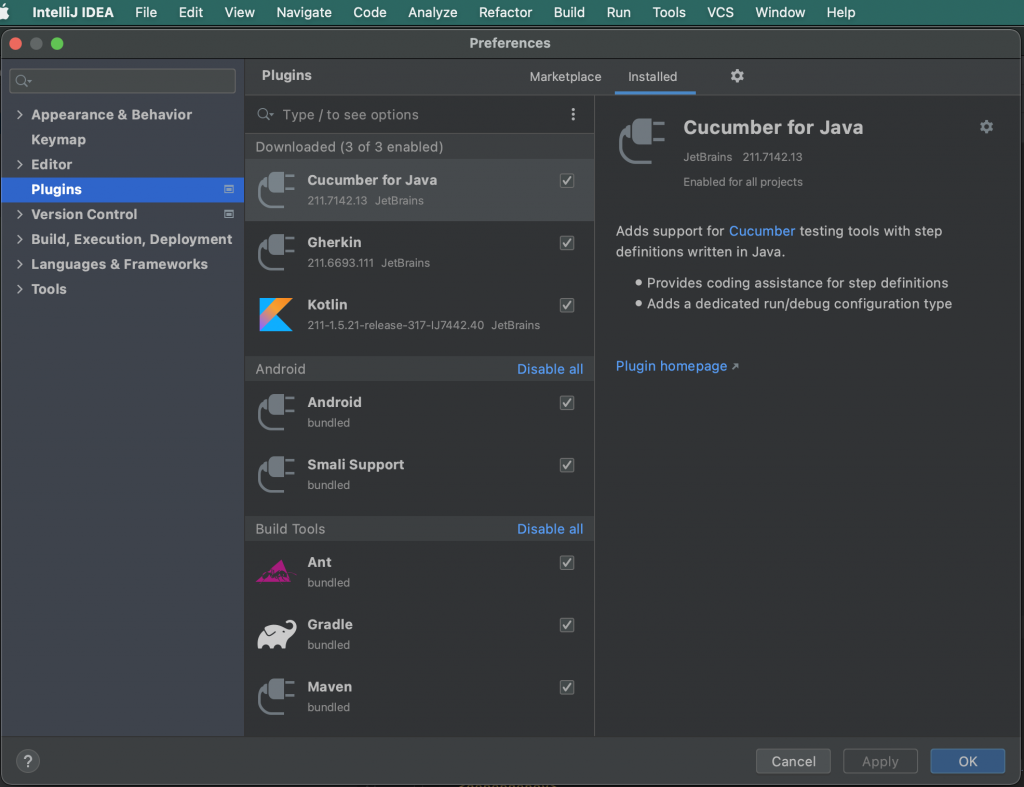
If you can’t find the plugins in the list, you may also type the name in the search bar. Search for the name of the plugins.
- Cucumber for Java
- Gherkin
- Maven
- JUnit
Create your first Maven project. Go to File > New Project > Maven > Next. Then Give your Maven project a Name and click on Finish button.
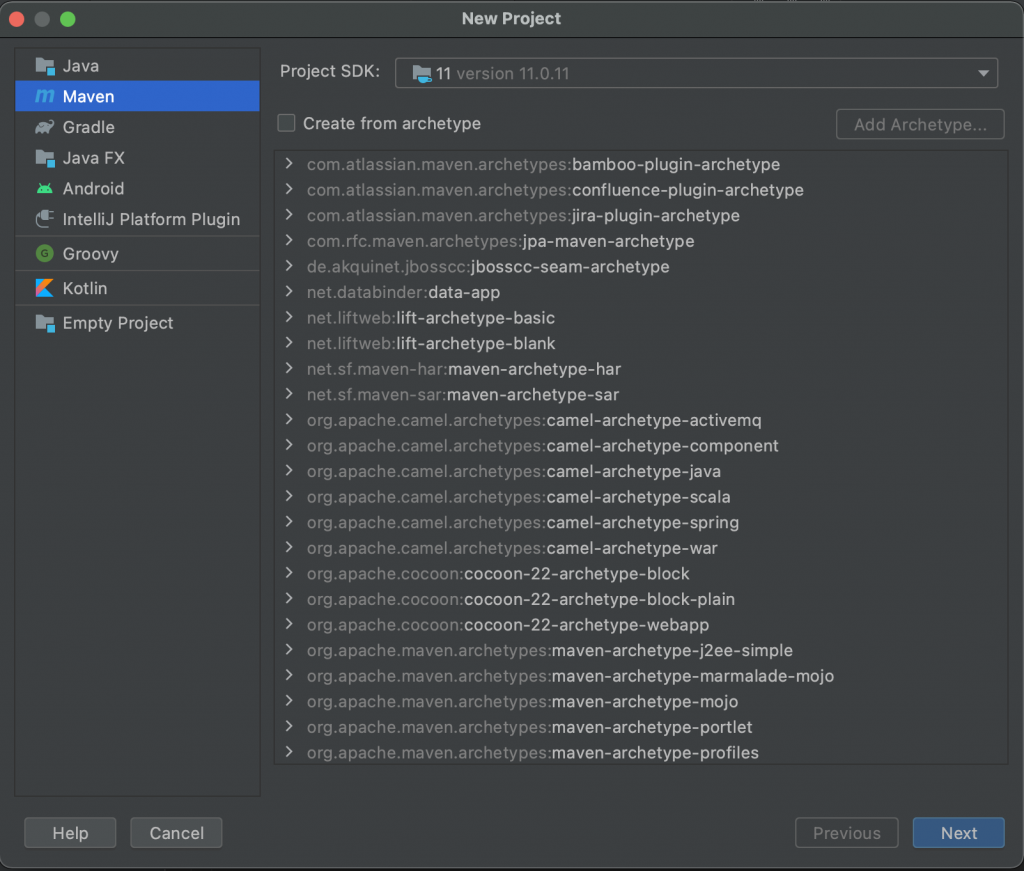
Alternatively, you can add all your test framework dependencies in pom.xml
file that will be added after you generate your fist Maven project.
Search for other dependency frameworks in Maven repository. Copy the entire <dendency>
XML and paste it in pom.xml
, inside the <dependencies>
.
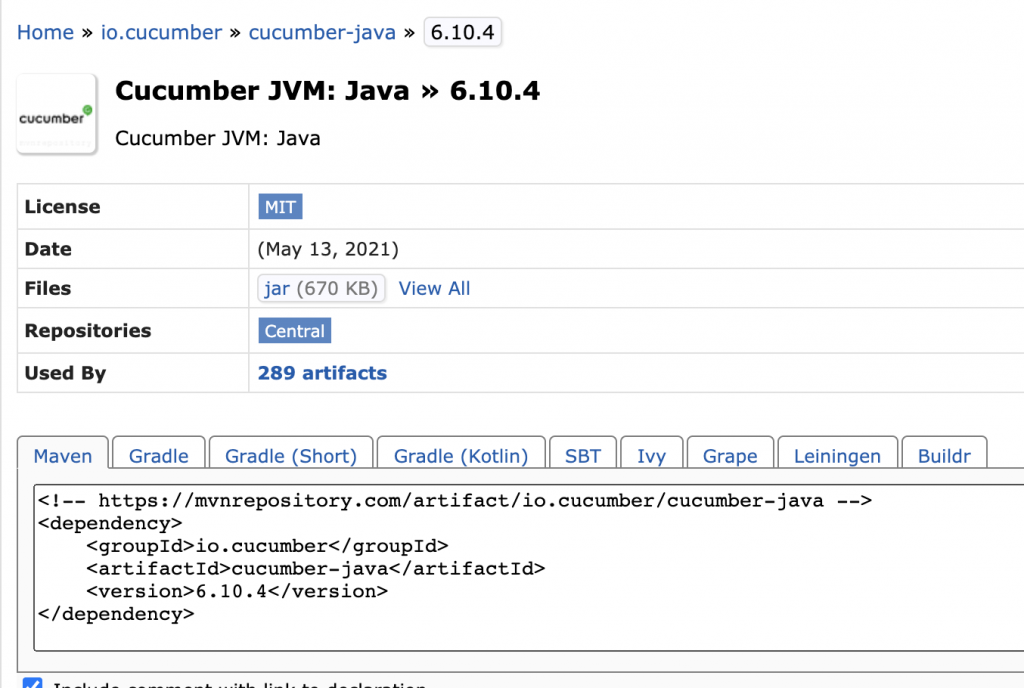
In case pom.xml
complain about dependency not found error, go to File > Invalidate Caches. This action will restart your IntelliJ.
Install Apache Maven (not the IntelliJ plugin). Using brew in MacOS
Maven is an important tool in Java for managing the build life cycle of your project. Their website provides a central repository for managing project JARS. It helps to maintain a common structure for your project. And provides commands that allow you to integrate CI tools like Jenkins for test automation.
The easy way to install Maven is by using brew.
brew install maven mvn -v
If you are having trouble with the installation using brew, you can also do a manual installation of Maven. Download the Maven JAR and follow the steps on how to add the bin directory to your PATH environment variable.
Run your test suite in Maven
To run your tests using the Maven commands, you need to add the surefire plugin. Copy the tag into pom.xml
file.
<pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>3.0.0-M5</version> </plugin> </plugins> </pluginManagement>
Integration testing useful Maven commands
Here are some of the useful commands in Maven for managing your project’s test suite.
Generate a new Maven project through CLI.
mvn archetype:generate -B -DgroupId=com.rex_maven.example -DartifactId=MyFirstMaven -DarchetypeArtifactId=maven-archetype-quickstart -Dversion=0.1
You can find more options for defining system properties using the -D
tag. The archetype is for defining the structure of your project and in this example we used the basic structure maven-archetype-quickstart.
Attempt to clean the files and directories generated by Maven during its build. Deletes the target folder.
mvn clean
Compile the source code of the project and checks for syntax errors. However, It does not actually run the test.
mvn compile
Run all tests using a suitable unit testing framework. Also, it will attempt to compile if it has not been compiled yet.
mvn test
Sometimes, you may need to just run a single test or you need to trigger your test suite through a test runner class. This is also possible by specifying this in a tag. For example, I have a JUnit test runner ApiTestRunner
to trigger all my cucumber tests.
package org.example.runner; import io.cucumber.junit.Cucumber; import io.cucumber.junit.CucumberOptions; import org.junit.runner.RunWith; @RunWith(Cucumber.class) @CucumberOptions( features = "src/test/java/org/example/features", glue = "org.example.steps", tags = "@hello_world", stepNotifications = true, plugin = {"pretty", "html:target/cucumber.html", "junit:target/cucumber.xml", "json:target/cucumber-report.json"}, monochrome = true ) public class ApiTestJunitRunner { }
Then I can trigger my test runner class in Maven.
mvn -Dtest=ApiTestRunner test
See more about running a single test in Maven.
Maven compile error. Source option 6 is no longer supported. Use 7 or later
Make sure that you add the Maven compiler plugin to your pom.xml
. If you have not done this yet, go to the Maven plugin documentation and copy the tag for maven-compiler-plugin.
If you are using JDK version 11, apply the release version under configuration tag. Take note, in the older version you specify the source and target parameters. But in here you only need the release parameter.
<pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </pluginManagement>
In my next tutorial, I will be discussing on how to set up a Java Maven project for testing using Cucumber JUnit. I will have examples on how to run your tests directly in Cucumber feature files. And also, using JUnit test runner class.