Get Started With Angular – But Where Should You Start?
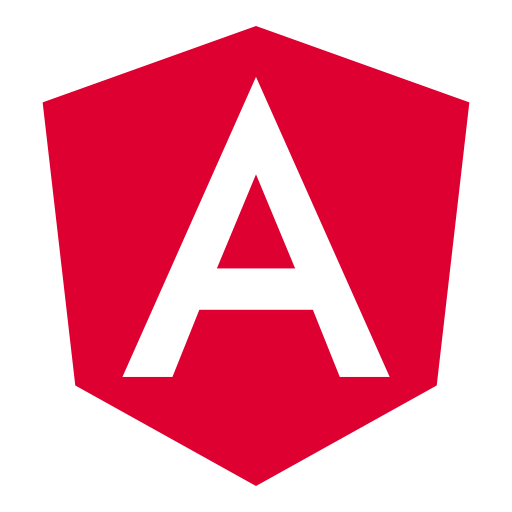
What is Angular?
Angular is a web application framework that you can use to develop apps on multiple platforms. It works for web, mobile web, native mobile and native desktop. Angular is the successor of AngularJS (or some call it Angular 1).
Angular files Typescript extension (.ts)
Files in Angular are saved using the “.ts” extension, because it is actually written in Typescript programming language, a strict syntactical superset of Javascript.
When you run your application, the files are then transpiled back into Javascript which is the language that browsers will understand. Angular CLI has some built in tools that can convert your files to Javascript by just running a single command. Read the guide in Angular.io about compiler options.
Run this command to build your app for production.
ng build --prod
Is it required that you know how to code in Typescript?
When I first built my project in Angular, it helped me a lot to understand the core API of the framework and write the parts of my application (Component, Service, Pipe, and so on) by learning at least the basics of Typescript.
It is not required, but I recommend to have a decent understanding and you should be confident to code in Typescript. Having said that, do not get overwhelmed or discouraged if you have not used the language before. After all, Typescript is a superset of Javascript, therefore existing Javascript program is also a valid Typescript program. Learning Typescript should not be too hard as you may think (you will probably enjoy it).
Angular vs jQuery
Both are NOT comparable. In fact, you can use jQuery library inside Angular framework, and if you do not have jQuery installed, Angular use its own jQlite, a subset of jQuery.
If you come from a jQuery background (like me), you have to detach your mindset from its simple premise of building a page first then attaching DOM events to manipulate the content.
Understand Angular From a Different View
The best way to condition yourself is to think of the Model, View, Controller (MVC) approach. This approach is common in back-end frameworks, where you define your object first in a Model and use the definition inside your Controller to process the data, and finally calling your view to render the page.
Angular uses Component (this is called Controller in AngularJS) and HTML is then associated with a Component Class. Unlike in jQuery where you have to manually trigger the DOM events, the data updates the view automatically through its two-way binding mechanism.
For example, I have a property “posts” in my Component Class “PostComponent”. After retrieving the posts data from the database, the div element below will execute a loop to display the contents. With two-way binding, I can edit,delete, and add posts that will automatically change the view.
<div *ngFor="let post of posts" > <p>({{post.id}}) {{post.title}}</p> </div>
Do I have to learn AngularJS before jumping to Angular?
AngularJS is the early version of Angular. It is not necessary to learn AngularJS, In fact it is a separate project and it has already entered the final stretch of its Long Term Support. It is not a bad idea to read about AngularJS, but I recommend building your future project using the newest version of Angular.
Where should I Start to Learn Angular?
It could be overwhelming in the beginning, but as soon as you get the hang of it you will actually enjoy building the dynamic blocks of your page using Angular. To get started with Angular, I suggest the following below (in order):
- Read about Node.js and npm to manage all the packages you will need, including an install of angular-cli
- Learn Typescript. You do not have to master the language, at least go over their TypeScript in 5 minutes tutorial.
- Get familiar with Angular app structure. From the root app, routing to each page. Understand the concept of Single-page Application.
- Start practicing. The best way to learn is by doing it yourself, checkout the Angular Tour of Heroes App tutorial.
This will not make you an expert overnight, even for an experienced front-end developer, it takes time to fully learn all the features Angular has to offer. But the purpose of this is to get your hands dirty and start somewhere .
Checkout Example Codes
- Angular Class and Style Binding
- Angular CLI generate sub module and its component
- How to install specific version of nodejs, npm and angular-cli
- Built in Pipes
- Template-driven Forms
- Example use Observable Part 1
- Example use Observable Part 2
- HTTP Get Post Put Delete Part 1
- Manage versions of node npm angular cli
- HTTP Get Post Put Delete Part 2
- HTTP Get Post Put Delete Part 3