AWS Lambda function
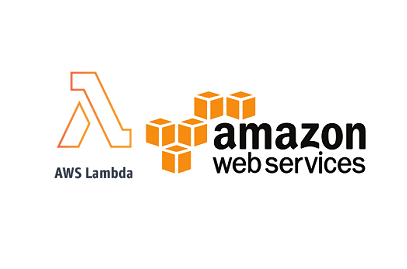
What is AWS Lambda and Function as a Service (FaaS)? How to create an AWS Lambda function?
What is AWS Lambda and FaaS
AWS Lambda is part of the serverless architecture and fully managed services by Amazon. Function as a Service (Faas) on the other hand, is a platform that deploy and run application functionalities.
FaaS became popular for Developers especially when building microservices applications because of its efficiency, ideal for CI/CD and it eliminates the complexity of managing a host server.
In addition, Lambda is also useful when facilitating the integration between AWS services. For instance, receiving request from an API Gateway and saving the data into DynamoDB.
How to create a Lambda
First, login to AWS Management Console and in the Dashboard search for “Lambda”.

You will see on the top right corner the options to select the region from where do you want to host your Lambda function. Also on the top right corner, click on “Create Function” button.
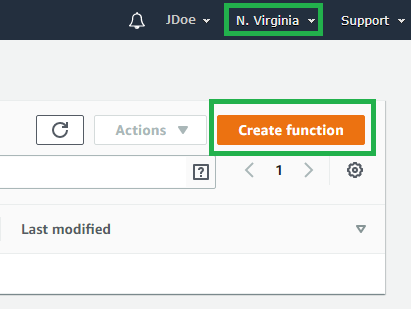
Inside the Lambda creation form, you can choose how you want to create your function, this could be from scratch, use a blueprint or browse from the app repository.

For this tutorial, we will choose Author from scratch to demo the Lambda. Then we can give our function a name and select the environment that will run our codes. Also we will create a new IAM Role with basic Lambda permissions.
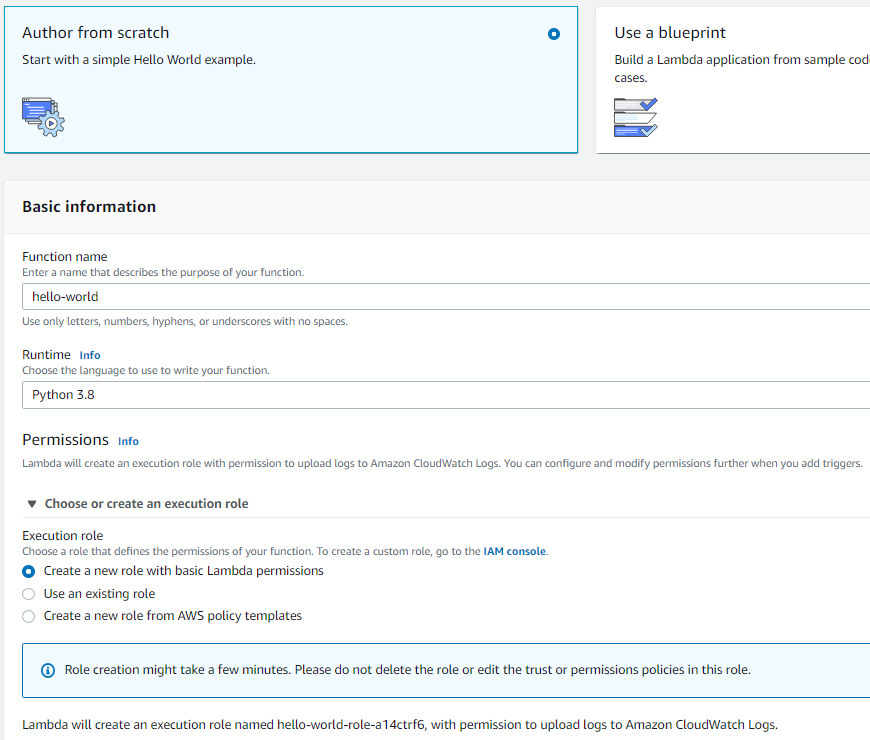
Once you have created your Lambda function, you will be able to manage its configuration, permissions, view monitoring, submit a test and write your function codes.
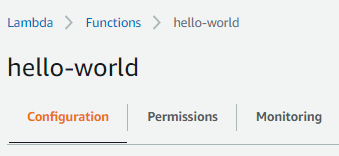

By default, Lambda will create a file with a simple function handler as an example and you can add more files inside the package as needed.
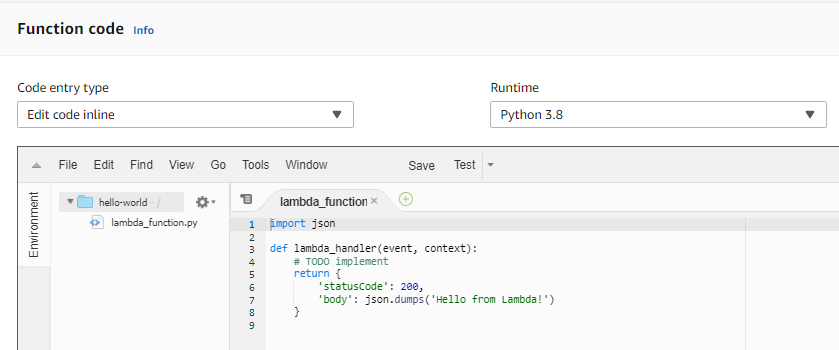
For more advanced code entry type, you can upload a .zip file from your local computer or from Amazon S3. These setup are useful when your function has dependency libraries.
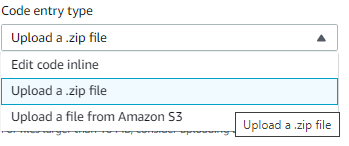
It is also important to mention that you need to define your function handler in the configuration. This will serve as an entry point to your Lambda application.
For example, if you have a module called lambda_function and inside is the function lambda_handler, you can specify the import path in the Handler setting to be lambda_function.lambda_handler.
def lambda_handler(event, context): ...
Import path in Handler setting.
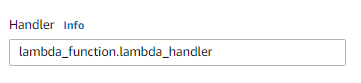
Attach CloudWatch log policy into Lambda
For this demo, we will print the event parameter and return the value of “key1”. To display the output, first we will have to give our Lambda the permission to read and write logs in CloudWatch.
Under permissions tab, click on the Role name, then you will get redirected to the IAM Roles and choose to attach policies.
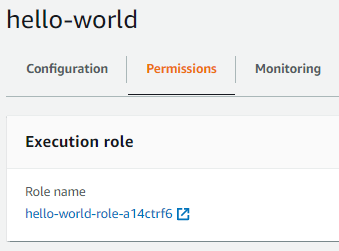
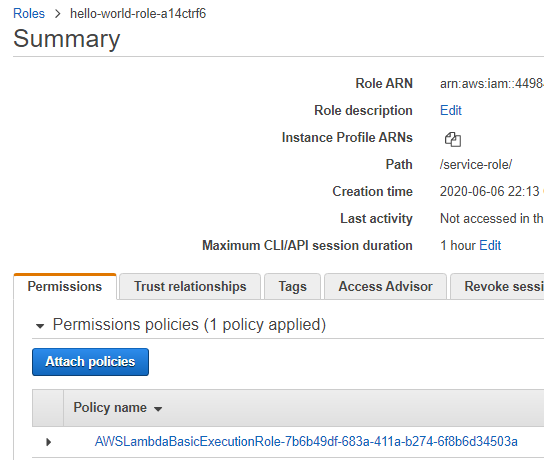
In the Filter policies field, search for “cloudwatchlogs”, then select the policy from the search results.
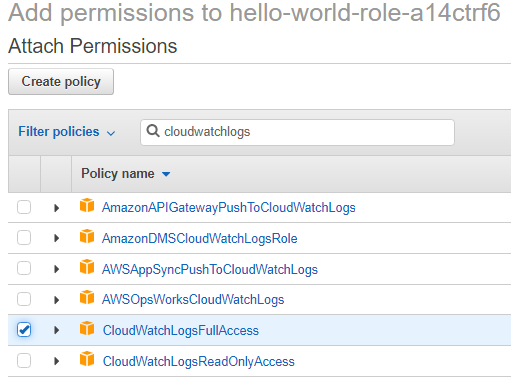
Test Lambda function
After attaching the new policy, we can go back to the Lambda and write our code to return the value of the event parameter.
import json def lambda_handler(event, context): print(event) return { 'statusCode': 200, 'body': json.dumps(event["key1"]) }
To test this we need to configure the test event and give it a name. Also you will notice that the value of our test event is in JSON format, with a key named “key1”.
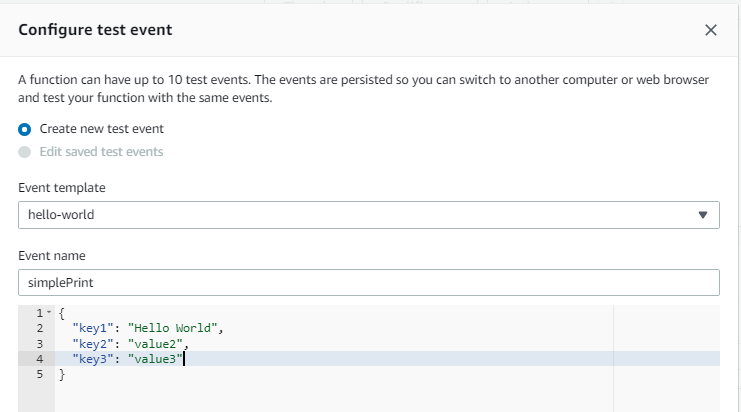
Running the Test and we should see the Execution result. Since it succeeded, we can view the value of “key1” as the result returned in our function execution.
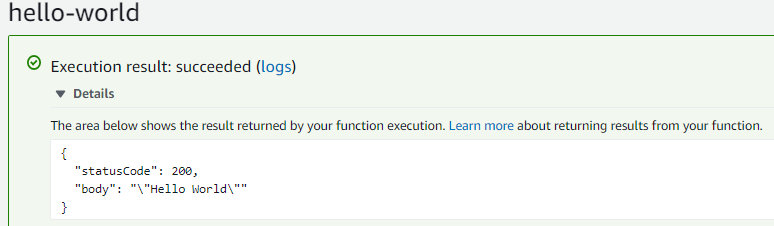
Moreover, we can view the printed logs in CloudWatch. This is useful if you are trying to debug something in your codes.
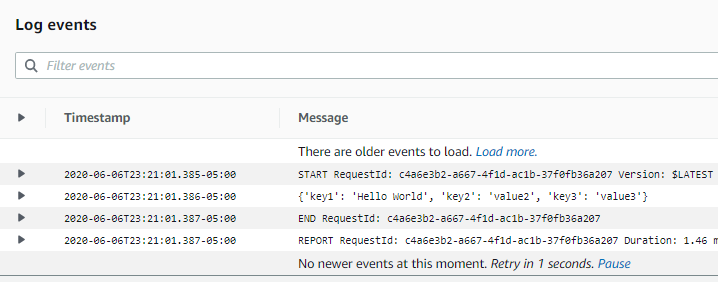